Permalink
- Free Seating Chart Software
- Classroom Seating Chart Program
- Seating Chart Program Java Download
- Seating Chart Program Java Free
- Seating Chart Program Java Pdf
JAVA PROBLEM A theater seating chart is implemented as a two-dimmension array of ticket prices like this: int[][] seats - Answered by a verified Tutor. A theater seating chart is implemented as a two-dimmension array of ticket prices like this. I have a java programming assignment that requires multiple programs to be written One is a.
Free Seating Chart Software
Join GitHub today
GitHub is home to over 40 million developers working together to host and review code, manage projects, and build software together.
Sign up/*A theater seating chart is implemented as a two-dimensional array of ticket prices, |
like this: |
10 10 10 10 10 10 20 20 30 10 |
10 10 10 10 10 20 30 40 10 10 |
10 20 20 20 30 30 50 10 10 10 |
20 20 20 30 40 50 10 10 10 20 |
20 20 40 50 50 10 10 10 20 20 |
20 40 50 50 10 10 10 20 20 20 |
30 40 50 10 10 10 20 20 20 30 |
30 50 10 10 10 10 10 10 20 30 |
40 10 10 10 10 10 10 20 20 30 |
Write a program that prompts users to |
pick either a seat or a price. Mark sold |
seats by changing the price to 0. When |
a user specifies a seat, make sure it is |
available. When a user specifies a price, |
find any seat with that price.*/ |
importjava.util.Scanner; |
publicclassP6_24 { |
publicstaticvoidmain(String[] args) { |
int[][] prices =newint[][] { |
{ 10, 10, 10, 10, 10, 10, 20, 20, 30, 10 }, |
{ 10, 10, 10, 10, 10, 20, 30, 40, 10, 10 }, |
{ 10, 20, 20, 20, 30, 30, 50, 10, 10, 10 }, |
{ 20, 20, 20, 30, 40, 50, 10, 10, 10, 20 }, |
{ 20, 20, 40, 50, 50, 10, 10, 10, 20, 20 }, |
{ 20, 40, 50, 50, 10, 10, 10, 20, 20, 20 }, |
{ 30, 40, 50, 10, 10, 10, 20, 20, 20, 30 }, |
{ 30, 50, 10, 10, 10, 10, 10, 10, 20, 30 }, |
{ 40, 10, 10, 10, 10, 10, 10, 20, 20, 30 } |
}; |
Scanner input =newScanner(System.in); |
System.out.print('Please enter (seat or price): '); |
String choose = input.next(); |
while (!(choose.equals('seat') || choose.equals('price'))) { |
System.out.print('Please enter (seat or price): '); |
choose = input.next(); |
} |
boolean isSold =false; |
while (!isSold) { |
if (choose.equals('seat')) { |
System.out.print('Please choose row: '); |
int row = input.nextInt(); |
System.out.print('Please choose column: '); |
int column = input.nextInt(); |
if (prices[row][column] !=0) { |
isSold =true; |
prices[row][column] =0; |
System.out.println('This seat is empty! You got it!'); |
} else { |
System.out.println('This seat is not empty! Pick another one!'); |
} |
} elseif (choose.equals('price')) { |
System.out.print('Plese choose price: '); |
int price = input.nextInt(); |
for (int i =0; i < prices.length; i++) { |
for (int j =0; j < prices[i].length; j++) { |
if (prices[i][j] price) { |
isSold =true; |
prices[i][j] =0; |
System.out.printf('This seat [%d, %d] is empty! You got it!n', i, j); |
break; |
} |
} |
if (isSold) { |
break; |
} |
} |
if (!isSold) { |
System.out.println('Please pick another price!'); |
} |
} |
} |
System.out.println('Goodbye! Have a nice day!'); |
input.close(); |
} |
} |
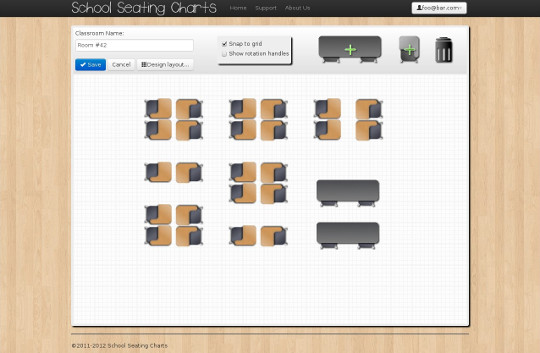
Copy lines Copy permalink
Let me first say, this is a 'Lab' that I'm doing for class, and I've been working on this second of two programs for around 5 hours now, probably 2 of them stuck on this one part. Let me first give you the requirements of the program so that maybe all of this will make a bit more sense.
A theater seating chart is implemented as a two-dimensional array of ticket prices, like this:
Write a program that prompts users to pick either a seat or a price. Mark sold seats by changing the price to 0. When a user specifies a seat, make sure it is available. When a user specifies a price, find any seat with that price.
That's the book's description, here's what my professor has given me to go with as well.
Required Program Components: The initial seating chart in this solution is generated as shown in the example. The solution also assumes that the user will enter a row and seat which start at 1 and where row 1 is the bottom front row. Your program should have at least 3 static methods that i) print the array, ii) sellSeatByPrice, and iii)sellSeatByNumber. Please declare and initialize your array to the provided seat price values in main.
What I've gotten myself stuck on for around 2 hours is figuring out how to change just one of the numbers of the 2D array to a 0. Say I entered a seat for 30, I've changed my code around 5 times now to get it to work and I'll either have every 30 change to a 0, or a few of them, or none. The main portion I need help on is the method called sellSeatByPrice(). Currently I'm not getting any numbers to change. Here's my current full code:
(I do apologize if this looks sloppy, it's 3am and I can't think anymore)
Classroom Seating Chart Program
2 Answers
First, you check found true
(which can be shortened to if (found)
, by the way). found
is initialized to false
and is never touched again, so the check fails every time.
You don't even need a boolean variable here. Iterate over the seats; if a seat has the price you're looking for, set the seat's price to 0, print the array and return (break
only exits the inner for
loop).